MobX has a pretty small API surface and tries to get out of your way as much as possible. Although minimal, there are few concepts youshould be familiar with. This guide is meant to be a crash course in MobX.
Flutter also offers many ready to use widgets (UI) to create a modern application. These widgets are optimized for mobile environment and designing the application using widgets is as simple as designing HTML. To be specific, Flutter application is itself a widget. Flutter widgets also supports animations and gestures. Displaying PDF from Network, File and assets easily like we display Image in Flutter Widget. Repository (GitHub) View/report issues. Flutter, fluttercachemanager, http, pathprovider. Packages that depend on pdfflutter.
Required Packages
Make sure to have the following packages installed for working effectively with MobX
and Flutter
:
The main MobX package that includes Observables
, Actions
, and Reactions
Provides the Observer
widget that auto-renders when the tracked observables change.
A powerful code-generator that greatly improves the developer experience with MobX. It provides annotations like@observable
, @computed
, @action
which hides all the boilerplate in a separately generated file, *.g.dart
.
These packages should appear in your pubspec.yaml
like below.
Declaring a Store class
Every store in MobX should be declared with the following boilerplate. This is probably the only boilerplate thatgets repeated. You could make this into a code-snippet in your IDE. Below, we are declaring a Todo
store.
Note that the basename of the part-file must match the containing-file exactly! In the above case, the partfile is called todo.g.dart
, which matches the todo.dart
file in which it is contained. To generate the part-file,you have to run the following command:
Ya, it looks like a mouthful 🙃 but it does the job!
Adding @observable, @computed, @action
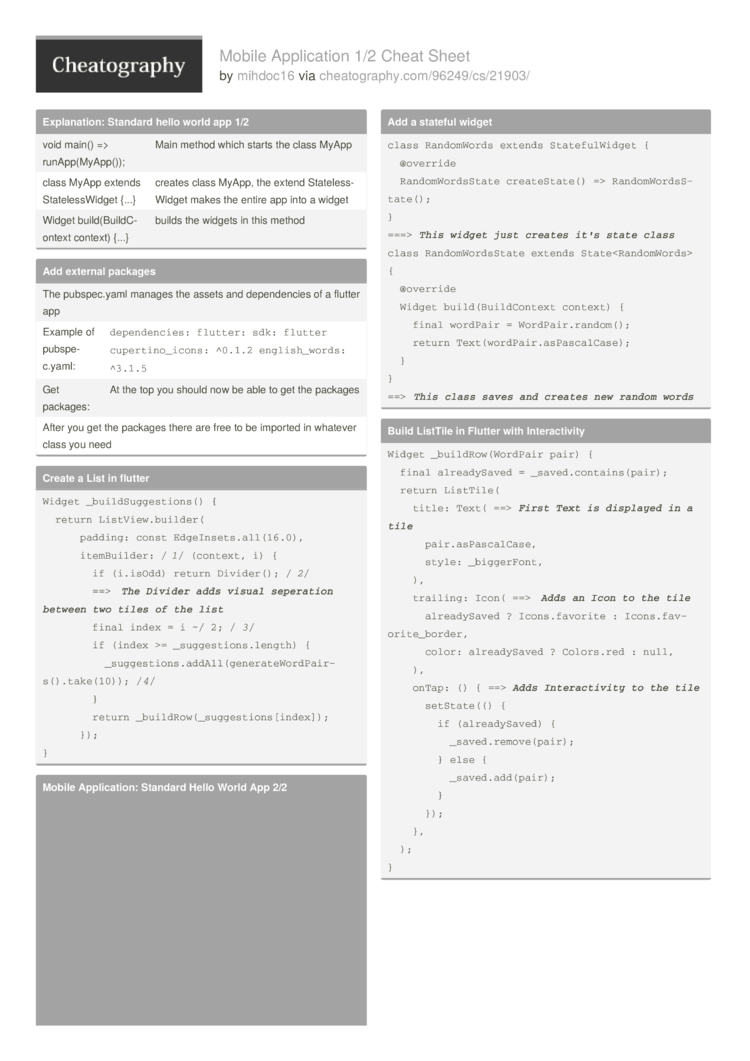
Flutter Widgets Cheat Sheet Pdf
Observables are the reactive state of your store and Actions are semantic operations that mutate them. ComputedObservables are read-only properties that depend on other observables and auto-update when any of the dependentobservables change.
Reactive wrappers
MobX also comes with a set of wrapper-classes that add the reactive behavior. These include:

ObservableList<T>
ObservableSet<T>
ObservableMap<K,V>
ObservableFuture<T>
ObservableStream<T>
Reactive Extensions
You can convert plain List
, Map
, Set
, Future
and Stream
instances into an observable version with the asObservable()
extension method.For example, in the code-snippet above, you could do:
Don't underestimate the @computed
Although @computed
looks like a simple, readonly observable, it can easily be your most powerful tool.By creating @computed
properties that depend on other observables, you can dramatically simplify the UI code andeliminate most of the business logic inside your Widgets. It is most often used for hiding conditional logic andcalculating some derived information.

For example, rather than checking if some data is loaded successfully inside a Widget...
...you can create a @computed
property called hasResults
...
Flutter Layout Widgets
...and simplify your widget logic...
...Since a @computed
property is an observable, the Observer
will automatically render when it changes!
Adding reactions

Flutter Widget Cheat Sheet
Reactions, as the name suggests, react to changes in observables. Without reactions, it would be a boring systemthat only produces changes in observables but nothing visible or useful ever happens because there are no reactions!
There are 3 types of reactions: autorun
, reaction
, when
. Each reaction returns a ReactionDisposer
, which when called will disposethe reaction. Disposing a reaction stops tracking the observables.
Flutter Layout Cheat Sheet
autorun
: Is a long-running reaction and starts tracking immediately.
reaction
: Is a long-running reaction and starts tracking only after the first change. It runs the effect whenany tracked observables change.
Flutter Stateful Widget Cheat Sheet
when
: a reaction that waits for a condition to become true before running the effect. After running the effect,it automatically disposes. Thuswhen
is a one-time only reaction!.
